Welcome to the world of Selenium testing! If you’re an automation tester, you know that creating a script is only half the battle. The real challenge comes when you need to debug and fix issues that are preventing your script from running as expected.
Debugging is a crucial skill for any automation tester, and it’s something that separates the best from the rest. Just like a movie director fine-tunes every shot, you, too, can perfect your automation tests with the right tools and techniques.
However, debugging can sometimes feel like a game of detective work. You need to employ some savvy techniques to get to the bottom of any issues. But don’t worry, with the right approach and the right tools, you can find and fix even the most elusive bugs.
In this article, we’ll take you through some advanced debugging techniques that will help you take your Selenium scripts to the next level. We’ll show you how to use cutting-edge debugging strategies to identify and solve issues that might be preventing your tests from running successfully. So, get ready to learn how to debug your Selenium scripts like a pro.
Advanced Techniques For Debugging Selenium Test Scripts
Here are some advanced techniques for debugging Selenium test scripts:
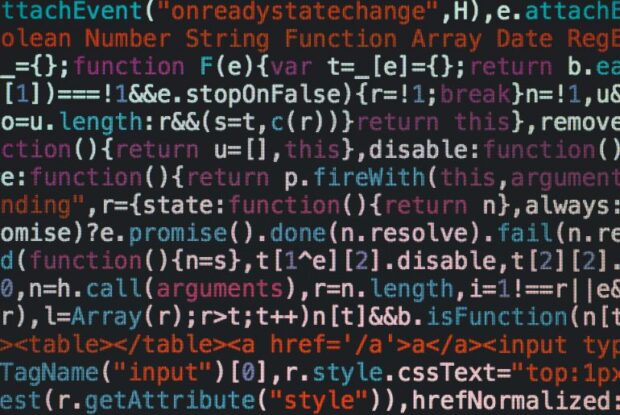
1. Debugging Selenium Tests on Already Opened Browser
Sometimes, you might want to debug your Selenium tests on a browser that is already open. Here are some examples:
- If you have a long test suite, opening and closing the browser for each test case can be time-consuming. By using an already open browser, you can save time by skipping the browser setup and teardown process.
- When you encounter a test failure, it can be helpful to investigate the error in real time on the same browser that the test was running on. By attaching your Selenium test to an already opened browser, you can see what happened on the page and potentially identify the cause of the failure more easily.
- You may want to test certain scenarios where the browser is already in a certain state, such as when a user is already logged in. In this case, you can manually open the browser, log in, and then attach your Selenium tests to the already open browser to test the desired scenario.
To do this, you can attach your Selenium WebDriver instance to the existing browser session by using the attachToSession() method. This can help you debug issues that might only occur in certain browser configurations.
WebDriver driver = new ChromeDriver();
String sessionID = driver.getSessionId().toString(); //Get the session ID
//Attach to the existing browser session
WebDriver driver = new RemoteWebDriver(new URL(“http://localhost:9515”), DesiredCapabilities.chrome());
((RemoteWebDriver)driver).setSessionId(sessionID);
This code allows you to connect to an existing WebDriver session and continue interacting with the browser using Selenium. This technique can be handy when you want to continue testing or interacting with the same browser instance without having to start a new one each time.
2. Session Recording
Session recording tools are software programs that can record all of the actions and events that happen while you run a Selenium test script. These recordings can help you to identify any issues or problems that may be occurring during testing, particularly if they are intermittent or difficult to reproduce.
For example, let’s say you are testing a web application with a Selenium test script. You run the script, and it appears to pass without any errors. However, when you review the test results, you notice that there are some unexpected issues that are occurring intermittently. These issues are not easily reproducible, so you’re not sure what’s causing them.
With a session recording tool, you can record the entire testing session and review it later to see what happened during the test. This can help you spot any issues that occurred during the test that you might have missed during manual testing. You can also use the recording to analyze the test results in more detail and identify any patterns or trends that may be causing the issues.
There are several session recording tools available, such as:
- Selenium Video Node: https://github.com/Ardesco/Selenium-Maven-Template
- Selenoid: https://aerokube.com/selenoid/latest/
- Zalenium: https://github.com/zalando/zalenium
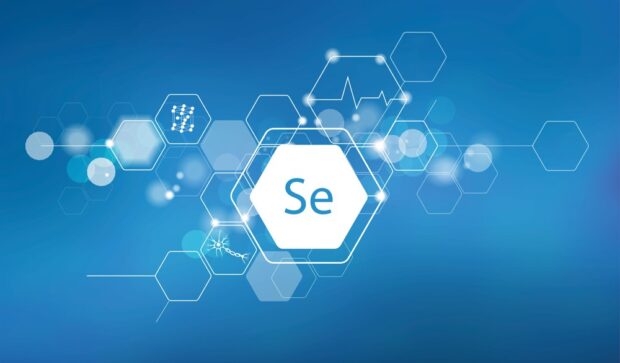
3. Adding Breakpoints
A breakpoint is a debugging tool used by developers to pause the execution of a running program at a specific line of code. By adding a breakpoint at the print statement, the code execution will pause at that point, allowing the developer to inspect the state of the program and variables at that point in time. This can help you identify issues that might be causing the problem.
WebDriver driver = new ChromeDriver();
driver.get(“https://www.google.com”);
//Add a breakpoint to stop execution at a specific point
System.out.println(“Adding breakpoint”);
This code opens the Google homepage using Selenium WebDriver and adds a breakpoint for debugging purposes. The print statement is added as a marker to indicate where the breakpoint has been added.
4. Capturing Screenshots
Capturing screenshots of your test script as it runs can help you identify issues that might not be obvious from your code or logs. You can capture screenshots at specific points in your test script’s execution or when a particular condition is met.
To capture a screenshot of a web page using Selenium, you can use the getScreenshotAs() method of the TakesScreenshot interface. Here is an example of capturing a screenshot in Java:
WebDriver driver = new ChromeDriver();
// Navigate to a web page
driver.get(“https://www.example.com”);
// Capture a screenshot
File screenshot = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE);
// Save the screenshot to a file
FileUtils.copyFile(screenshot, new File(“screenshot.png”));
In this example, we first create a new ChromeDriver instance to control a Chrome browser window. We then navigate to the web page at https://www.example.com. Finally, we capture a screenshot of the current state of the browser window using the getScreenshotAs() method of the TakesScreenshot interface. This method returns a File object that represents the screenshot image. We can then save this file to disk using the FileUtils.copyFile() method from the Apache Commons IO library.
You can place this code at specific points in your test script to capture screenshots at those points. For example, you might capture a screenshot after submitting a form or after clicking a button to verify that the correct page or result is displayed.
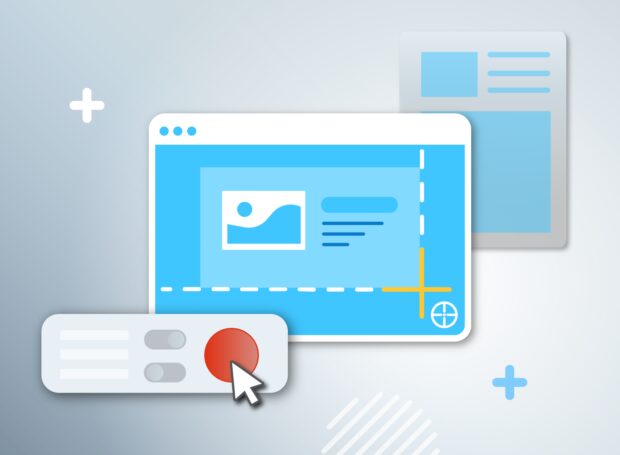
5. Debugging with Logging Utilities
Logging utilities such as log4j or logback can help you debug your Selenium test scripts at various levels of severity. By adding logging statements to your code, you can get insight into what’s happening at each step of your test script. This can help you identify where the issue is occurring and what’s causing it.
Here is an example of using log4j in Java:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class MyTest {
private static final Logger logger = LogManager.getLogger(MyTest.class);
public void myTestMethod() {
logger.debug(“Starting my test method”);
WebDriver driver = new ChromeDriver();
logger.debug(“Navigating to web page”);
driver.get(“https://www.example.com”);
//more code
logger.debug(“Finished my test method”);
}
}
In this example, we import the org.apache.logging.log4j package and create a Logger instance using the LogManager.getLogger() method. We can then use the logger.debug() method to write messages to the log file at the DEBUG level.
In the myTestMethod() method, we first write a debug message indicating that the method has started. We then create a new ChromeDriver instance and navigate to a web page. We can write another debug message to indicate that we have started navigating to the page. We can continue writing debug messages at various points in our test method to track its progress and identify any issues.
You can configure log4j to write log messages to a file or to the console, and you can adjust the log level to control which messages are written. By adding logging statements to your test script, you can gain valuable insight into what’s happening at each step and identify where issues are occurring.
6. Use LT(LambdaTest) Debug
LT Debug browser extension is a powerful debugging tool designed with the user in mind. One of the main benefits of LT Debug extension is that it is completely free of cost forever.
To get started with the LT Debug extension, you will need to fill out a simple form, and you will have access to powerful debugging tools right away. The extension is designed to be easy to use and intuitive, so you can start debugging your applications quickly and efficiently.
In addition to the LT Debug extension, you will also get access to over 100 other extensions that have been exclusively developed by and for developers and testers. This means that you will have access to a wide range of tools and resources that can help you streamline the debugging process and improve the overall quality of your applications.
Overall, the LT Debug extension is the perfect tool for anyone who wants to debug their applications quickly and easily. With its user-friendly interface, powerful features, and access to a wide range of other developer and tester tools, this extension is a must-have for anyone who takes debugging seriously.
Here is a step-by-step guide to installing LT Debug with a human touch:
Step 1: Open your Google Chrome browser and navigate to the LT Debug Chrome Extension page. You can easily find this page by typing “LT Debug Chrome Extension” in the search bar of your browser or by visiting the website https://www.lambdatest.com/lt-debug.
Step 2: Once you’re on the extension page, you will see a blue button labeled “Add to Chrome” in the top right corner. Click on it.
Step 3: You will now be redirected to the Chrome Web Store. Here, you will see the LT Debug extension page again, along with another blue button labeled “Add to Chrome”. Click on it.
Step 4: A pop-up will appear asking if you want to add “LT Debug” to your browser. Click on the “Add extension” button to confirm.
Step 5: Congratulations! You have now successfully installed LT Debug. You can access the extension by clicking on the puzzle icon in the top right corner of your browser, then clicking on the LT Debug icon.
Now that you have installed the extension, you can choose the feature you want to use and start debugging your code. Happy coding!
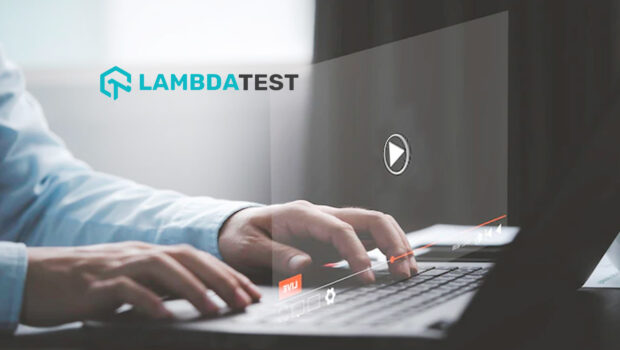
7. Code Review
Sometimes, it can be helpful to have someone else review your code to identify issues that you might have missed. A fresh set of eyes could often spot issues that you might have overlooked.
Code review is the process of having someone else examine your code to identify issues, suggest improvements, and provide feedback. This is an important practice in software development because it can help catch errors and improve code quality.
For example, imagine you’re a developer who just finished writing a piece of code for a new feature in your application. You’re confident that the code works correctly, but you decide to have a colleague review it before submitting it for testing. Your colleague takes a look at your code and notices a few areas where the code could be improved, and suggests a better approach to implement the feature. Additionally, they find some errors that you missed, such as a potential null pointer exception, and provide guidance on how to fix them.
By having someone else review your code, you can benefit from their fresh perspective and knowledge, and they may spot issues or provide insights that you didn’t consider.
Eliminating the Hassle of Debugging with LambdaTest
As a developer or tester, you know how frustrating it is to spend hours or even days debugging a website or application. Finding and fixing issues can be time-consuming and stressful, especially when working with different browsers and devices.
You may have tried using different tools to debug your website, but they all come with their limitations. The solution to this problem is LambdaTest. With this solution, you can test your website or application on 3000+ different browsers and devices in real time. This means you can inspect and debug issues as they occur, eliminating the need for long debugging sessions. Additionally, you can also take screenshots, record videos, and perform other actions to help you understand the issue better.
LambdaTest is easy to use and requires no additional setup. All you need is a LambdaTest account and an internet connection. You can choose from a variety of configurations, including different browsers, devices, versions, OS, screen resolutions, and more.
Step 1: First, you’ll need to sign up and log in to your LambdaTest account. Once you’re logged in, head over to the Real Time Testing section, which you can find in the left-hand navigation menu. Here, you’ll be able to choose the test configuration you’d like to use, including the browser, device, version, operating system, screen resolution, and more. This will help ensure that your website looks and functions properly across a variety of platforms.
Step 2: Once you’ve selected your test configuration, it’s time to provide the URL of the website you’d like to debug. This could be your own website or someone else’s that you’re working on. Simply enter the URL into the provided field and hit enter.
Step 3: Next, it’s time to start inspecting the website. To do this, simply right-click your mouse pointer and choose the “Inspect” option. This will open up a new window where you can start exploring the website’s code and design elements.
Step 4: Finally, you can begin inspecting the website as per your needs. This might include looking for errors in the code, checking the layout and design, or testing various features and functions to make sure everything is working as it should.
Say goodbye to the frustration of debugging and say hello to LambdaTest!
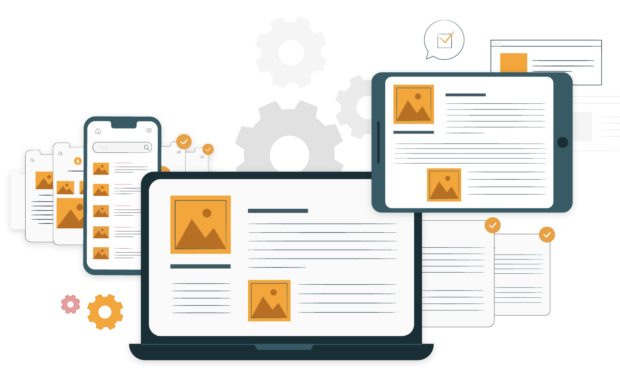
Wrap-up
As we come to the final end of our journey through the world of advanced techniques for debugging Selenium test scripts, it’s time to take a moment and reflect on what we’ve learned.
When we encounter a stubborn bug in our test script, it can be tempting to give up and move on to something else. But with the right tools and techniques at our disposal, we can keep chipping away at the problem until we finally emerge victorious.
In the world of testing, what really matters is that we approach debugging with a positive attitude and willingness to learn and adapt. Just like the characters in our favorite movies and cartoons, we can overcome any obstacle if we put our minds to it. So let’s get out there and debug those scripts like the heroes!